How to upload YouTube Videos Effortlessly with Rust Programming
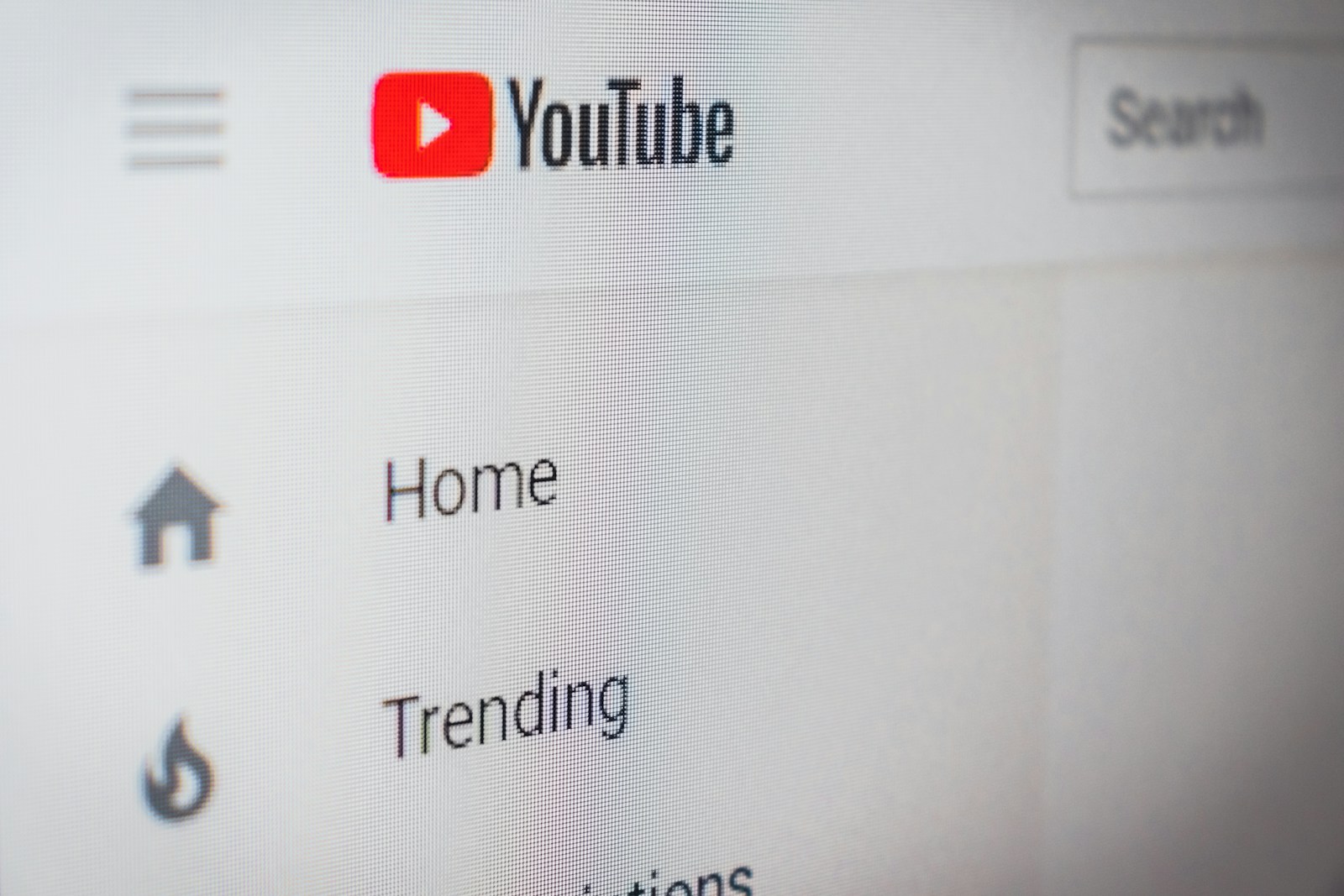
Welcome, Rustaceans and YouTube creators! Have you ever considered the power of Rust for automating your YouTube uploads? Rust’s reliability and efficiency make it a superb choice for interacting with YouTube’s API. This guide will take you through every step of using Rust to upload videos to YouTube, complete with detailed instructions and ready-to-use sample code. Whether you’re new to Rust or looking to extend your project’s capabilities, you’re in the right place to learn about automating YouTube uploads with Rust.
Setting Up Your Rust Environment
Before diving into code, ensure your Rust environment is set up. If you’re new to Rust, start by installing the Rust compiler and Cargo (Rust’s package manager and build system) from the official Rust website. Once installed, you can create a new project by running:
cargo new youtube_uploader
cd youtube_uploader
This command sets up a new Rust project called “youtube_uploader” and moves you into the project directory.
Installing Necessary Dependencies
To interact with the YouTube API and handle HTTP requests efficiently in Rust, we need to include a couple of essential crates in our project: google_youtube3
for YouTube API operations and hyper
for making HTTP requests. You can setup these dependencies by running:
cargo add google_youtube3 hyper hyper-rustls tokio oauth2 serde serde_json # for adding dependencies
cargo build # for building dependencies
Understanding OAuth2 for YouTube API Authentication
To upload videos to YouTube via their API, you need to authenticate your application using OAuth2. The provided code snippet kicks off this process by creating an oauth2::ApplicationSecret
instance with your client credentials.
Ensure you have a project set up in the Google Developers Console, enable the YouTube Data API v3, and obtain your client_id
and client_secret
. These values are essential for the OAuth2 flow.
Implementing YouTube Video Uploads in Rust
Now, let’s dive into the heart of uploading videos to YouTube with Rust, using the code snippet you provided as a foundation. Let’s first import all dependencies we need for our script:
use google_youtube3::{
api::{Scope, Video, VideoSnippet, VideoStatus},
hyper, hyper_rustls, oauth2, YouTube,
};
use hyper::Body;
use hyper_rustls::HttpsConnector;
use log::info;
use oauth2::hyper::client::HttpConnector;
use std::path::Path;
OAuth2 Authentication Setup:
The code begins by creating an oauth2::ApplicationSecret
with your client credentials. This object is then used to build an InstalledFlowAuthenticator
for handling the OAuth2 flow, including user authorization and token management.
let secret: oauth2::ApplicationSecret = oauth2::ApplicationSecret {
client_id: "YOUR_CLIENT_ID",
client_secret: "YOUR_CLIENT_SECRET",
auth_uri: "https://accounts.google.com/o/oauth2/auth".to_string(),
token_uri: "https://oauth2.googleapis.com/token".to_string(),
..Default::default()
};
let auth = oauth2::InstalledFlowAuthenticator::builder(
secret,
oauth2::InstalledFlowReturnMethod::HTTPRedirect,
)
.build()
.await.unwrap();
Make sure to replace the client_id
and client_secret
fields respectively.
Creating the YouTube API Client:
Next, we instantiate a YouTube
client using the Hyper library for making HTTP requests, configuring it for both HTTPS and HTTP/1.
let hub = YouTube::new(
hyper::Client::builder().build::<_, Body>(
hyper_rustls::HttpsConnectorBuilder::new()
.with_native_roots()
.https_or_http()
.enable_http1()
.build(),
),
auth,
);
Preparing and Uploading the Video:
You’ll need to specify the path to the video file you want to upload. The video is then prepared with metadata such as the title and description, followed by the actual upload process.
let video_file = std::fs::File::open("YOUR_VIDEO_PATH").unwrap();
let video = Video {
snippet: Some(VideoSnippet {
title: Some("Test Video Title".to_string()),
description: Some("This is a test video uploaded via the YouTube API.".to_string()),
..Default::default()
}),
status: Some(VideoStatus {
privacy_status: Some("private".to_string()), // This will upload the video private
..Default::default()
}),
..Default::default()
};
let (_, video) = hub
.videos()
.insert(video)
.add_scope(Scope::Upload)
.upload(video_file, "video/*".parse().unwrap())
.await.unwrap();
Make sure to update the video path you are reading the video file from.
Handling Common Issues
- Async/Await: The code uses asynchronous Rust, so your main function must be asynchronous as well. You can use the
tokio
runtime to execute your async code. - Error Handling: Make sure to handle potential errors at each step, especially during the OAuth flow and the video upload process.
Wrapping Up
By following this guide and utilizing the provided sample code, you’re now equipped to automate YouTube video uploads using Rust. This approach not only enhances your productivity but also leverages Rust’s robustness and safety features.
As you become more comfortable with Rust and the YouTube API, consider exploring additional functionalities such as updating video metadata, managing playlists, and analyzing channel analytics.
Happy coding, and may your videos reach a wide audience on YouTube!
Taking Your Automation Further
With your newfound ability to automate YouTube uploads using Rust, why stop there? Imagine the possibilities if you could extend your automation skills to other platforms and applications. If you’ve ever considered automating interactions on WhatsApp or integrating AI-powered conversations with a tool like ChatGPT, we’ve got just the guide for you.
Expand Your Horizons with a Golang WhatsApp ChatGPT Bot: Ready to explore more? Check out our comprehensive guide on Creating a ChatGPT Bot for WhatsApp with Golang. This guide will walk you through the steps of using Golang to build a bot that can interact with users on WhatsApp, powered by the capabilities of ChatGPT. Whether it’s for fun, customer support, or something else, the applications are endless. Dive in and start building today!
Share this content:
Leave a Reply