How to integrate ChatGPT with WhatsApp using Golang Part 2
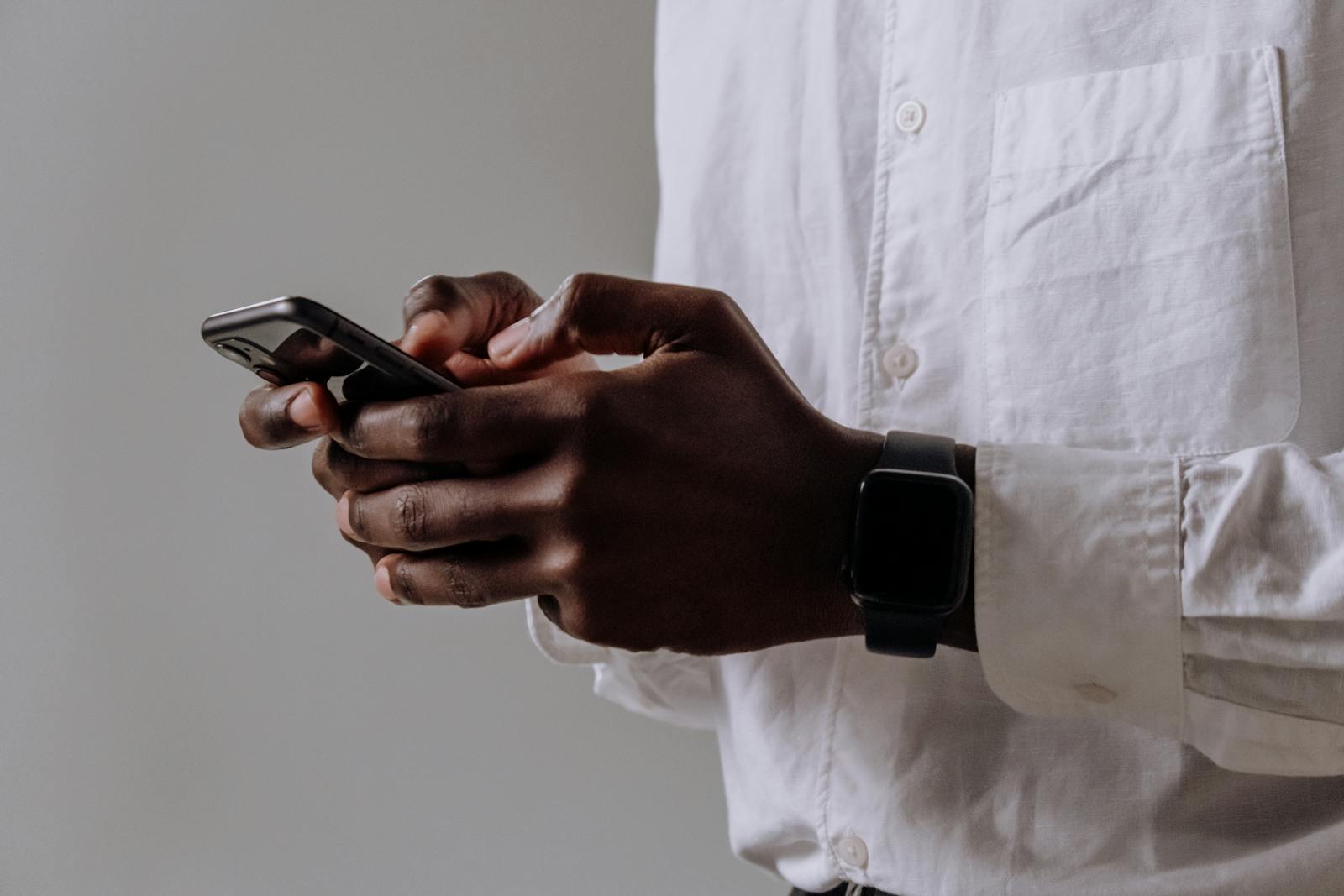
Part1: https://www.mindevice.net/whatsapp-chatgpt-part1
In this second part of the tutorial, we will cover how to handle incoming messages and send responses using the OpenAI ChatGPT API.
Handling Incoming Messages
First we need to create a function that let’s us handle message events
import (
"go.mau.fi/whatsmeow/types/events"
)
func HandleEvent(evt interface{}) {
switch v := evt.(type) {
case *events.Message:
go HandleMessage(v)
}
}
func HandleMessage(messageEvent *events.Message) {
// Handle Messages here
}
The above code includes a HandleEvent
function that takes an event as input and uses a switch statement to determine the type of the event. If the event is of type *events.Message
, it calls the HandleMessage
function asynchronously.
Now we need to add the HandleEvent
function to the client event handlers. To do this, we need to update the main
function like this:
func main() {
WhatsmeowClient := CreateClient()
ConnectClient(WhatsmeowClient)
WhatsmeowClient.AddEventHandler(HandleEvent);
}
Now your WhatsApp Bot should be able to listen to Message Events.
Setting up OpenAI API
First we need to store the OpenAI API key as an environment secret. To do this create a .env
file.
# .env
OPENAI_API_KEY=sk...
To create a OpenAI API Client from the secrets create the following function
func GetOpenAIClient() (*openai.Client, error) {
err := godotenv.Load()
if err != nil {
return nil, err
}
apiKey := os.Getenv("OPENAI_API_KEY")
client := openai.NewClient(apiKey)
return client, nil
}
The above code loads the environment variable and create a new OpenAI API cilent.
Now we need to create a global OpenAI Client to be able to use it from everywhere inside our code.
var OpenAIClient *openai.Client
func main() {
client := CreateClient()
ConnectClient(client)
client.AddEventHandler(HandleEvent)
OpenAIClient, _ = GetOpenAIClient()
}
Sending Responses
Now we are ready to send responses. For that we need update the HandleMessage
function appropriately. First we need to get the content of the message.
import (
waProto "go.mau.fi/whatsmeow/binary/proto"
)
func HandleMessage(messageEvent *events.Message) {
messageContent := messageEvent.Message.GetConversation()
resp, err := OpenAIClient.CreateChatCompletion(
context.Background(),
openai.ChatCompletionRequest{
Model: openai.GPT3Dot5Turbo,
Messages: []openai.ChatCompletionMessage{
{
Role: openai.ChatMessageRoleUser,
Content: messageContent,
},
},
},
)
if err != nil {
return
}
reply := resp.Choices[0].Message.Content
WhatsmeowClient.SendMessage(context.Background(), messageEvent.Info.Chat, &waProto.Message{
Conversation: &reply,
})
}
First we get the content of our message. After that we generate a chat completion using OpenAI ChatGPT API. After that we send a message back with the corresponding response.
Now you should be ready to have a conversation with ChatGPT from WhatsApp!

The repo of the above tutorial can be found here:
https://github.com/SushiWaUmai/whatsapp-chatgpt-tutorial/
Also we have made an extended version of the WhatsApp bot with more features!
https://github.com/SushiWaUmai/prince/
Share this content:
Leave a Reply